In enterprise applications, caching plays a vital role in optimizing performance by reducing the load on databases and improving response times. One widely used caching solution is JCache, which provides a standardized API for integrating caching into Java applications. However, developers sometimes encounter issues when using ProxyJCacheConfiguration, which can lead to unexpected system failures or performance bottlenecks.
This article explores the common causes of failure in ProxyJCacheConfiguration and offers a step-by-step solution to fix the problem. By following these best practices, you can ensure a stable and efficient caching implementation in your Spring-based application.
Understanding the Issue
The issue with ProxyJCacheConfiguration often arises when Spring Boot applications attempt to use JCache annotations such as @Cacheable
, @CachePut
, or @CacheEvict
incorrectly. This usually happens due to misconfigurations, class-proxying conflicts, or reliance on unsupported cache providers.
Common symptoms of this failure include:
- Caching does not work: Your application does not store or retrieve cached values as expected.
- Unexpected exceptions: Errors related to proxy creation or cache resolution occur at runtime.
- Performance degradation: The application experiences latency due to improper cache handling.
Root Causes of ProxyJCacheConfiguration Failure
Several factors may contribute to failures in ProxyJCacheConfiguration. Here are the most common causes:
- Missing or Incorrect JCache Configuration: The application does not properly define a supported cache manager, leading to misconfigurations.
- Spring Proxy Mechanism Conflicts: JCache annotations require Spring’s proxy-based mechanism, which may not function correctly due to class proxying limitations.
- Incompatible Cache Provider: Not all cache providers fully support JCache annotations, causing unexpected behavior.
- Lack of Required Dependencies: Essential JCache dependencies, such as Ehcache, Hazelcast, or Infinispan, might be missing or outdated.
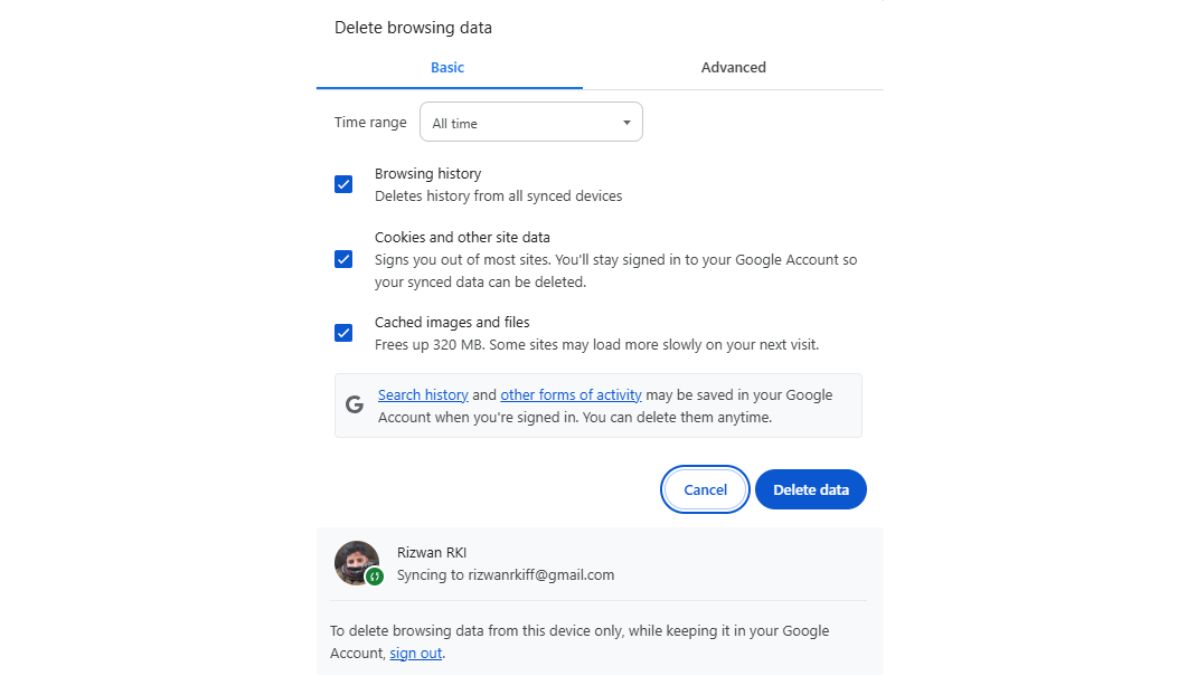
How to Solve ProxyJCacheConfiguration Failure
To resolve this issue, follow these steps:
1. Verify Dependencies
Ensure that your project has the necessary dependencies for both Spring Boot and JCache. If you are using Ehcache, for example, include the following dependencies in your pom.xml
(for Maven):
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>javax.cache</groupId>
<artifactId>cache-api</artifactId>
<version>1.1.1</version>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.10.0</version>
</dependency>
2. Enable Caching in Spring Boot
Ensure that your main application class is annotated with @EnableCaching
to activate Spring’s caching functionality:
@SpringBootApplication
@EnableCaching
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
3. Use Interface-Based Proxies
Spring uses proxies to implement caching functionality. If your class is not implementing an interface, Spring will create CGLIB-based proxies, which might cause conflicts. A best practice is to define an interface.
public interface MyService {
@Cacheable("myCache")
String fetchData(String key);
}
@Service
public class MyServiceImpl implements MyService {
@Override
public String fetchData(String key) {
return "Data for " + key;
}
}
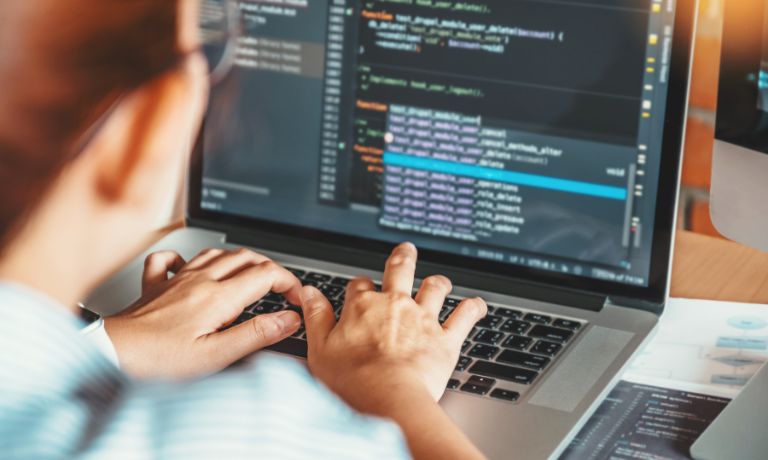
4. Define Cache Configuration Explicitly
In some cases, providing an explicit configuration for JCache can resolve conflicts. You can define a CacheManager
bean in a configuration class:
@Configuration
public class CacheConfig {
@Bean
public JCacheManagerCustomizer cacheManagerCustomizer() {
return cacheManager -> cacheManager.createCache("myCache",
new MutableConfiguration<>()
.setStatisticsEnabled(true)
.setExpiryPolicyFactory(AccessedExpiryPolicy.factoryOf(Duration.ONE_HOUR)));
}
}
Testing and Verification
Once you have applied these fixes, test the caching behavior using unit tests or by running the application and monitoring cache hits and misses. You can enable logging for cache operations to verify proper functionality:
logging.level.org.springframework.cache=DEBUG
If you still encounter issues, ensure that the correct cache implementation is being used by checking the application logs for cache manager initialization details.
Conclusion
Failures related to ProxyJCacheConfiguration can be frustrating, but by carefully troubleshooting the issue and following best practices, you can get JCache working smoothly in your Spring Boot application. Ensuring that dependencies are correct, using interface-based proxies, and explicitly defining cache configurations can help resolve most caching-related errors.
By implementing these solutions, you can optimize application performance and ensure that caching delivers the intended benefits efficiently and reliably.
I’m Sophia, a front-end developer with a passion for JavaScript frameworks. I enjoy sharing tips and tricks for modern web development.